You can display a few things in a search suggestion: the product name, image, SKU, a short description, and the price. Sometimes it’s not enough. If you sell wine, you may want to display the producer. If you sell books, you might want to display the author.
The following guide introduces a few tricks on how to display extra things like custom fields, attributes, or custom taxonomies in the search suggestions.
Table of Contents
Implementation (don’t skip it)
Below you will find some examples of snippets. Some of them must be running in a special WordPress SHORTINIT
mode and some as normal WordPress snippets. Here’s how to differentiate between them:
Running snippets
If the snippet includes [SHORTINIT MODE]
in the comment:
- Create a new empty
fibosearch.php
file in the root directory of your child theme and paste the snippet there.
If the snippet doesn’t include [SHORTINIT MODE]
:
- Apply it like a normal WordPress snippet e.g. in the
functions.php
file in your child theme or via the Code Snippets plugin.
Hooks to display custom content
Every search suggestion has 5 places you can use to display custom content. See the image below:
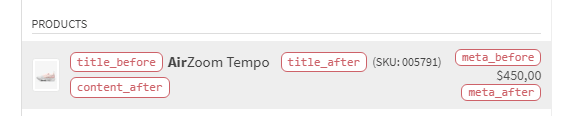
As you can see, there are the following hooks:
title_before
title_after
content_after
meta_before
meta_after
You can use the following filters to add extra info to products, variations, posts, pages or taxonomies:
dgwt/wcas/tnt/search_results/suggestion/product
: for productsdgwt/wcas/tnt/search_results/suggestion/product_variation
: for product variations (since FiboSearch 1.28.0)dgwt/wcas/tnt/search_results/suggestion/post
: for posts (since FiboSearch 1.28.0)dgwt/wcas/tnt/search_results/suggestion/page
: for pages (since FiboSearch 1.28.0)dgwt/wcas/tnt/search_results/suggestion/tax_{tax_slug}
: for taxonomies (since FiboSearch 1.28.0)
Note: post, page and taxonomy filters do not support the content_after
, meta_before
and meta_after
hooks.
Here is some sample code that adds extra text after a product name:
<?php // [SHORTINIT MODE] Run this snippet in the SHORTINIT mode (fibosearch.php file). Take a look at the Implementation section above. add_filter( 'dgwt/wcas/tnt/search_results/suggestion/product', function ( $data ) { $data['title_after'] = 'This text will be displayed next to product title'; return $data; });
ⓘLearn how to add this snippet in the special SHORTINIT mode.
Here’s another example for product categories:
<?php // [SHORTINIT MODE] Run this snippet in the SHORTINIT mode (fibosearch.php file). Take a look at the Implementation section above. add_filter( 'dgwt/wcas/tnt/search_results/suggestion/tax_product_cat', function( $data ) { $data['title_before'] = '(title_before)'; return $data; } );
ⓘLearn how to add this snippet in the special SHORTINIT mode.
Real-life examples
There are 3 snippets you can use for different cases. Use them as samples. Remember to replace the taxonomy name or custom field.
[Example 1] – Display book authors (custom taxonomy)
The book store wants to display authors in the search suggestions. They created a custom taxonomy book_author
and assigned books to the terms of this taxonomy.
Step 1 – Add book authors to the search index
This snippet allows you to save book authors in the memory, which will come in useful later for searching.
add_filter( 'dgwt/wcas/tnt/indexer/readable/product/data', function ( $data, $product_id, $product ) { $term = $product->getTerms( 'book_author', 'string' ); if ( ! empty( $term ) ) { $html = '<span class="suggestion-book-author">'; $html .= $term; $html .= '</span>'; $data['meta']['book_author'] = $html; } return $data; }, 10, 3 );
ⓘLearn how to add this snippet to your WordPress.
Step 2 – Rebuild the search index
Go to WooCommerce
→ FiboSearch
→ Indexer (tab)
and rebuild the search index. Wait for this process to finish.
Step 3 – Display authors while searching
This snippet allows you to read the book’s author from the storage while searching and then display it in the place of content_after
. This snippet has to be added to the special fibosearch.php
file. Scroll up to the Implementation section, and read about running snippets in SHORTINIT
WordPress mode.
<?php // [SHORTINIT MODE] Run this snippet in the SHORTINIT mode (fibosearch.php file). Take a look at the Implementation section above. add_filter( 'dgwt/wcas/tnt/search_results/suggestion/product', function ( $data, $suggestion ) { if ( ! empty( $suggestion->meta['book_author'] ) ) { $html = '<div class="suggestion-book-author">'; $html .= $suggestion->meta['book_author']; $html .= '</div>'; $data['content_after'] = $html; } return $data; }, 10, 2 );
ⓘLearn how to add this snippet in the special SHORTINIT mode.
[Example 2] – Display EAN code (custom field)
In this example, the EAN code is stored as a custom field called ean
. We want to display it below the product name.
Step 1 – Add the EAN code to the search index
This snippet allows you to save the EAN code in the memory, which will come in handy later for searching.
add_filter( 'dgwt/wcas/tnt/indexer/readable/product/data', function ( $data, $product_id, $product ) { $value = $product->getCustomField( 'ean' ); if ( ! empty( $value ) ) { $data['meta']['ean'] = $value; } return $data; }, 10, 3 );
ⓘLearn how to add this snippet to your WordPress.
Step 2 – Rebuild the search index
Go to WooCommerce
→ FiboSearch
→ Indexer (tab)
and rebuild the search index. Wait for this process to finish.
Step 3 – Display the EAN code while searching
This snippet allows you to read the EAN code from the storage while searching and then display it in the place of content_after
.
<?php // [SHORTINIT MODE] Run this snippet in the SHORTINIT mode (fibosearch.php file). Take a look at the Implementation section above. add_filter( 'dgwt/wcas/tnt/search_results/suggestion/product', function ( $data, $suggestion ) { if ( ! empty( $suggestion->meta['ean'] ) ) { $data['content_after'] = '<br />EAN: ' . $suggestion->meta['ean']; } return $data; }, 10, 2 );
ⓘLearn how to add this snippet in the special SHORTINIT mode.
[Example 3] – Display case pack size (product attribute)
In this example, we want to display the product size next to the product name. Size is a product attribute called size
. In WooCommerce, product attribute is just a taxonomy.
Step 1 – Find taxonomy name
The taxonomy name of an attribute has the form pa_{attribute_name}
. In our case, we have an attribute with slug case-pack-size
. This means that the taxonomy name is pa_case-pack-size
.
Alternatively, you can check the URL address when you are checking the attribute listing in the WordPress dashboard. Go to Products
→ Attributes
and select the attribute. Take a look at the URL address.

Step 2 – Follow the steps from [Example 1] – Display book authors
The next steps are the same as in Example 1. Just replace book_author
with your taxonomy.
[Example 4] – Display “in stock” or “out of stock” status
In this example, we want to display the stock status directly in the search suggestion.
Step 1 – Add the product stock status to the search index
This snippet allows you to save the product stock status in the memory, which will be useful later for searching.
add_filter( 'dgwt/wcas/tnt/indexer/readable/product/data', function ( $data, $product_id, $product ) { $value = $product->getStockAvailability(); if ( ! empty( $value ) ) { $data['meta']['stock'] = $value; } return $data; }, 10, 3 );
ⓘLearn how to add this snippet to your WordPress.
Step 2 – Rebuild the search index
Go to WooCommerce
→ FiboSearch
→ Indexer (tab)
and rebuild the search index. Wait for this process to finish.
Step 3 – Display stock status while searching
This snippet allows you to read stock availability from the storage while searching and then display it in the place of content_after
.
<?php // [SHORTINIT MODE] Run this snippet in the SHORTINIT mode (fibosearch.php file). Take a look at the Implementation section above. add_filter( 'dgwt/wcas/tnt/search_results/suggestion/product', function ( $data, $suggestion ) { if ( ! empty( $suggestion->meta['stock'] ) ) { $data['content_after'] = $suggestion->meta['stock']; } return $data; }, 10, 2 );
ⓘLearn how to add this snippet in the special SHORTINIT mode.
Step 4 – Add CSS for a better appearance
.dgwt-wcas-suggestion .dgwt-wcas-stock { margin: 3px 0 0 0; display: block; text-align: left; }
ⓘLearn how to add custom CSS to your WordPress.
Step 5 – Let’s see if it works
Check if the stock status shows up in the autocomplete. The results should look like this:
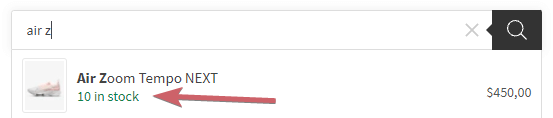
[Example 5] – Display product category description below categories
In this example, we want to display the product category description under the category in the search suggestions.
Step 1 – Add the category description to the search index
The following code will save the category description in the search index for later use.
add_filter( 'dgwt/wcas/indexer/taxonomy/insert', function ( $data, $termID, $taxonomy ) { $term = get_term_by( 'term_id', $termID, $taxonomy ); if ( ! is_array( $data['meta'] ) ) { $data['meta'] = []; } if ( ! empty( $term->description ) ) { $data['meta']['desc'] = wp_strip_all_tags( $term->description ); } return $data; }, 10, 3 );
ⓘLearn how to add this snippet to your WordPress.
Step 2 – Rebuild the search index
Go to WooCommerce
→ FiboSearch
→ Indexer (tab)
and rebuild the search index. Wait for this process to finish.
Step 3 – Display category description while searching
Add the following code to the fibosearch.php
file:
<?php // [SHORTINIT MODE] Run this snippet in the SHORTINIT mode (fibosearch.php file). Take a look at the Implementation section above. add_filter( 'dgwt/wcas/tnt/search_results/suggestion/tax_product_cat', function ($data) { if ( ! empty( $data['meta']['desc'] ) ) { $data['title_after'] = '<br><small>' . $data['meta']['desc'] . '</small>'; } return $data; } );
ⓘLearn how to add this snippet in the special SHORTINIT mode.
Step 4 – Let’s see if it works
Search for a category. The description should be displayed below it:
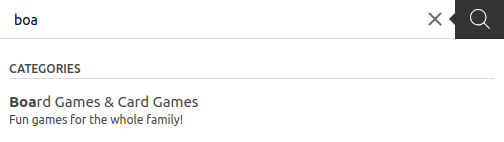